Complete Ethereum JSON-RPC List with examples
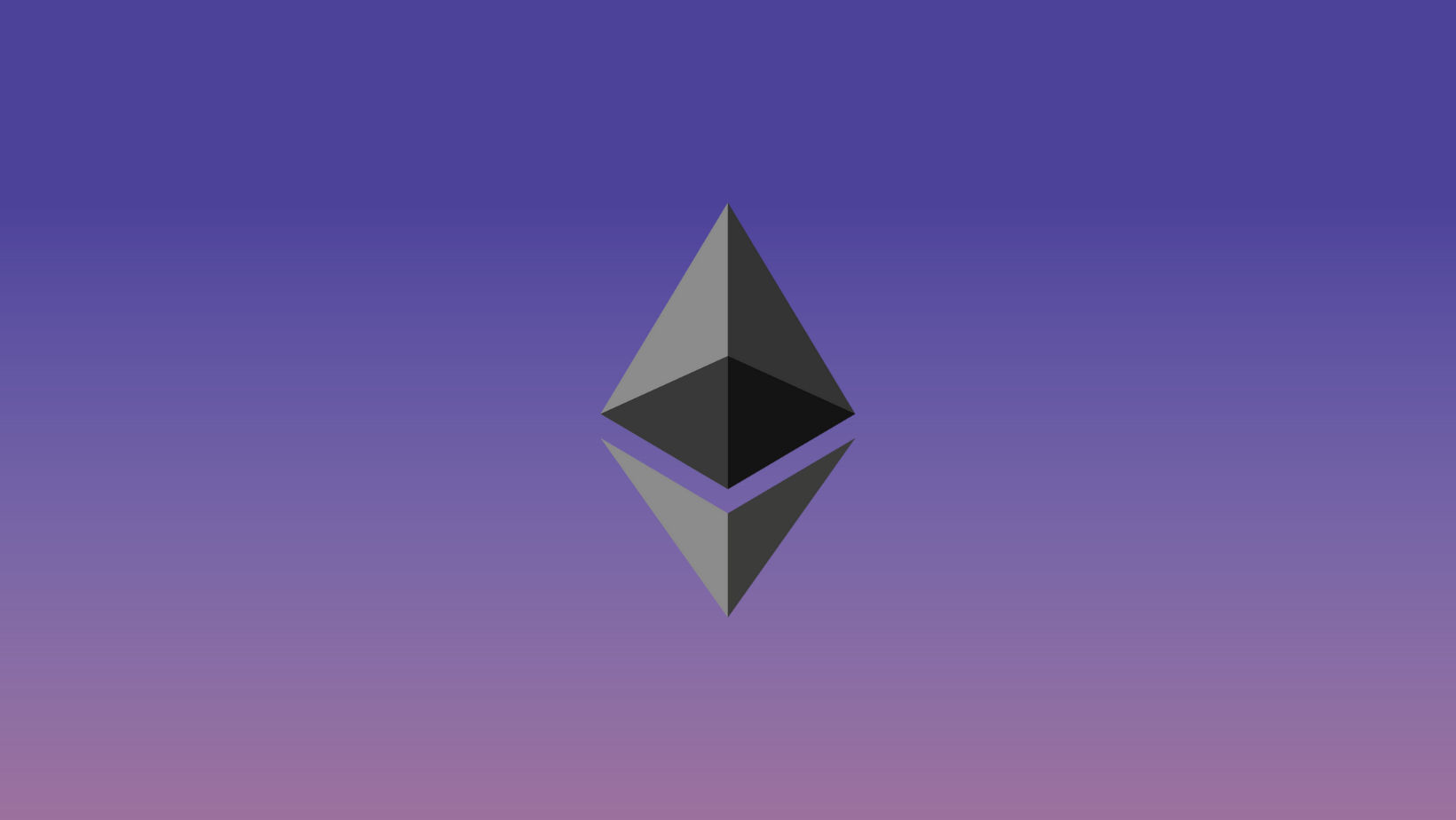
The following list of RPC calls and their examples are taken from eth.wiki & documenter.getpostman.com.
Ethereum JSON-RPC web3
clientVersion - Returns the current client version.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"web3_clientVersion",
"params":[],
"id":1
}'
sha3 - Returns Keccak-256 (not the standardized SHA3-256) of the given data.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"web3_sha3",
"params":["0x68656c6c6f20776f726c64"],
"id":64
}'
Ethereum JSON-RPC net
version - Returns the current network id.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"net_version",
"params":[],
"id":67
}'
listening - Returns true
if client is actively listening for network connections.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"net_listening",
"params":[],
"id":67
}'
peerCount - Returns number of peers currently connected to the client.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"net_peerCount",
"params":[],
"id":74
}'
Ethereum JSON-RPC eth
protocolVersion - Returns the current ethereum protocol version.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_protocolVersion",
"params":[],
"id":67
}'
syncing - Returns an object with data about the sync status or false.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_syncing",
"params":[],
"id":1
}'
coinbase - Returns the client coinbase address.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_coinbase",
"params":[],
"id":64
}'
mining - Returns true
if client is actively mining new blocks.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_mining",
"params":[],
"id":71
}'
hashrate -Returns the number of hashes per second that the node is mining with.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_hashrate",
"params":[],
"id":71
}'
gasPrice - Returns the current price per gas in wei.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_gasPrice",
"params":[],
"id":73
}'
accounts - Returns a list of addresses owned by client.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_accounts",
"params":[],
"id":1
}'
blockNumber - Returns the number of most recent block.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_blockNumber",
"params":[],
"id":83
}'
eth_getbalance - Returns the balance of the account of given address.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getBalance",
"params":[
"0x407d73d8a49eeb85d32cf465507dd71d507100c1",
"latest"
],
"id":1
}'
eth_getstorageat - Returns the value from a storage position at a given address.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method": "eth_getStorageAt",
"params": [
"0x295a70b2de5e3953354a6a8344e616ed314d7251",
"0x0",
"latest"
],
"id": 1
}'
eth_getTransactionCount - Returns the number of transactions sent from an address.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getTransactionCount",
"params":[
"0x407d73d8a49eeb85d32cf465507dd71d507100c1",
"latest"
],
"id":1
}'
eth_getBlockTransactionCountByHash - Returns the number of transactions in a block from a block matching the given block hash.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getBlockTransactionCountByHash",
"params":[
"0xb903239f8543d04b5dc1ba6579132b143087c68db1b2168786408fcbce568238"
],
"id":1
}'
eth_getBlockTransactionCountByNumber - Returns the number of transactions in a block matching the given block number.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getBlockTransactionCountByNumber",
"params":[
"0x52A8CA"
],
"id":1
}'
eth_getCode - Returns code at a given address.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getCode",
"params":[
"0xa94f5374fce5edbc8e2a8697c15331677e6ebf0b",
"0x2"
],
"id":1
}'
eth_sign - The sign method calculates an Ethereum specific signature with: sign(keccak256("\x19Ethereum Signed Message:\n" + len(message) + message)))
.
By adding a prefix to the message makes the calculated signature recognisable as an Ethereum specific signature. This prevents misuse where a malicious DApp can sign arbitrary data (e.g. transaction) and use the signature to impersonate the victim.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_sign",
"params":[
"0x9b2055d370f73ec7d8a03e965129118dc8f5bf83",
"0xdeadbeaf"
],
"id":1
}'
eth_sendTransaction - Creates new message call transaction or a contract creation, if the data field contains code.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_sendTransaction",
"params":[{
"from": "0xb60e8dd61c5d32be8058bb8eb970870f07233155",
"to": "0xd46e8dd67c5d32be8058bb8eb970870f07244567",
"gas": "0x76c0",
"gasPrice": "0x9184e72a000",
"value": "0x9184e72a",
"data": "0xd46e8dd67c5d32be8d46e8dd67c5d32be8058bb8eb970870f072445675058bb8eb970870f072445675"
}],
"id":1
}'
eth_sendRawTransaction - Creates new message call transaction or a contract creation for signed transactions.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_sendRawTransaction",
"params":["0xd46e8dd67c5d32be8d46e8dd67c5d32be8058bb8eb970870f072445675058bb8eb970870f072445675"],
"id":1
}'
eth_call - Executes a new message call immediately without creating a transaction on the block chain.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_call",
"params":[{
"from": "",
"to": "",
"gas": "",
"gasPrice": "",
"value": "",
"data": ""
}, "latest"],
"id":1
}'
eth_estimateGas - Generates and returns an estimate of how much gas is necessary to allow the transaction to complete. The transaction will not be added to the blockchain. Note that the estimate may be significantly more than the amount of gas actually used by the transaction, for a variety of reasons including EVM mechanics and node performance.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_estimateGas",
"params":[
],
"id":1
}'
eth_getBlockByHash - Returns information about a block by hash.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getBlockByHash",
"params":[
"0xad1328d13f833b8af722117afdc406a762033321df8e48c00cd372d462f48169",
true
],
"id":1
}'
eth_getBlockByNumber - Returns information about a block by block number.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getBlockByNumber",
"params":[
"0x1b4",
true
],
"id":1
}'
eth_getTransactionByHash - Returns the information about a transaction requested by transaction hash.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getTransactionByHash",
"params":[
"0xb2fea9c4b24775af6990237aa90228e5e092c56bdaee74496992a53c208da1ee"
],
"id":1
}'
eth_getTransactionByBlockHashAndIndex - Returns information about a transaction by block hash and transaction index position.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getTransactionByBlockHashAndIndex",
"params":[
"0x3c82bc62179602b67318c013c10f99011037c49cba84e31ffe6e465a21c521a7",
"0x0"
],
"id":1
}'
eth_getTransactionByBlockNumberAndIndex - Returns information about a transaction by block number and transaction index position.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getTransactionByBlockNumberAndIndex",
"params":[
"0x52A96E",
"0x1"
],
"id":1
}'
eth_getTransactionReceipt - Returns the receipt of a transaction by transaction hash.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getTransactionReceipt",
"params":[
"0xa3ece39ae137617669c6933b7578b94e705e765683f260fcfe30eaa41932610f"
],
"id":1
}'
eth_getUncleByBlockHashAndIndex - Returns information about a uncle of a block by hash and uncle index position.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getUncleByBlockHashAndIndex",
"params":[
"0x7cea0c9ae53df7073fcd4e7b19fc3f1905a2540bbdbd9a10796c9296f5af55dc",
"0x0"
],
"id":1
}'
getUncleByBlockNumberAndIndex - Returns information about a uncle of a block by number and uncle index position.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getUncleByBlockNumberAndIndex",
"params":[
"0x29c",
"0x0"
],
"id":1
}'
eth_getCompilers - Returns a list of available compilers in the client.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getCompilers",
"params":[],
"id":1
}'
eth_compileLLL - Returns compiled LLL code.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_compileLLL",
"params":[
"(returnlll (suicide (caller)))"
],
"id":1
}'
eth_compileSolidity - Returns compiled solidity code.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_compileSolidity",
"params":[
"contract test { function multiply(uint a) returns(uint d) { return a * 7; } }"
],
"id":1
}'
eth_compileSerpent - Returns compiled serpent code.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_compileSerpent",
"params":["/* some serpent */"],
"id":1
}'
eth_newFilter - Creates a filter object, based on filter options, to notify when the state changes (logs). To check if the state has changed, call eth_getFilterChanges
.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_newFilter",
"params":[
{
"topics":["0x12341234"]
}
],
"id":73
}'
newBlockFilter - Creates a filter in the node, to notify when a new block arrives. To check if the state has changed, call eth_getFilterChanges
.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_newBlockFilter",
"params":[],
"id":73
}'
newPendingTransactionFilter - Creates a filter in the node, to notify when new pending transactions arrive. To check if the state has changed, call eth_getFilterChanges
.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_newPendingTransactionFilter",
"params":[],
"id":73
}'
uninstallFilter - Uninstalls a filter with given id. Should always be called when watch is no longer needed. Additonally Filters timeout when they aren't requested with eth_getFilterChanges
for a period of time.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_uninstallFilter",
"params":[
"0xb"
],
"id":73
}'
getFilterChanges - Polling method for a filter, which returns an array of logs which occurred since last poll.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getFilterChanges",
"params":[
"0x16"
],
"id":73
}'
getLogs - Returns an array of all logs matching a given filter object.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getLogs",
"params":[{
"topics":[
"0x000000000000000000000000a94f5374fce5edbc8e2a8697c15331677e6ebf0b"
]
}],
"id":74
}'
getWork - Returns the hash of the current block, the seedHash, and the boundary condition to be met ("target").
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_getWork",
"params":[],
"id":73
}'
submitWork - Used for submitting a proof-of-work solution.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_submitWork",
"params":[
"0x0000000000000001",
"0x1234567890abcdef1234567890abcdef1234567890abcdef1234567890abcdef",
"0xD1GE5700000000000000000000000000D1GE5700000000000000000000000000"
],
"id":73
}'
submitHashrate - Used for submitting mining hashrate.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"eth_submitHashrate",
"params":[
"0x0000000000000000000000000000000000000000000000000000000000500000",
"0x59daa26581d0acd1fce254fb7e85952f4c09d0915afd33d3886cd914bc7d283c"
],
"id":73
}'
db
putString - Stores a string in the local database.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"db_putString",
"params":[
"testDB",
"myKey",
"myString"
],
"id":73
}'
getString - Returns string from the local database.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"db_getString",
"params":[
"testDB",
"myKey"
],
"id":73
}'
putHex - Stores binary data in the local database.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"db_putHex",
"params":[
"testDB",
"myKey",
"0x68656c6c6f20776f726c64"
],
"id":73
}'
getHex - Returns binary data from the local database.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"db_getHex"
,"params":[
"testDB",
"myKey"
],
"id":73
}'
shh
post - Sends a whisper message.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"shh_post",
"params":[{
"from":"0xc931d93e97ab07fe42d923478ba2465f2..",
"topics": [
"0x68656c6c6f20776f726c64"
],
"payload":"0x68656c6c6f20776f726c64",
"ttl":0x64,
"priority":0x64
}],
"id":73
}'
version - Returns the current whisper protocol version.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"shh_version",
"params":[],
"id":67
}'
newIdentity - Creates new whisper identity in the client.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"shh_newIdentity",
"params":[],
"id":73
}'
hasIdentity - Checks if the client hold the private keys for a given identity.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"shh_hasIdentity",
"params":[
"0x04f96a5e25610293e42a73908e93ccc8c4d4dc0edcfa9fa872f50cb214e08ebf61a03e245533f97284d442460f2998cd41858798ddfd4d661997d3940272b717b1"
],
"id":73
}'
newGroup
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"shh_newGroup",
"params":[],
"id":73
}'
addToGroup
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"shh_addToGroup",
"params":[
"0x04f96a5e25610293e42a73908e93ccc8c4d4dc0edcfa9fa872f50cb214e08ebf61a03e245533f97284d442460f2998cd41858798ddfd4d661997d3940272b717b1"
],
"id":73
}'
newFilter - Creates filter to notify, when client receives whisper message matching the filter options.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"shh_newFilter",
"params":[{
"topics": [
"0x12341234bf4b564f"
],
"to": "0x2341234bf4b2341234bf4b564f..."
}],
"id":73
}'
uninstallFilter - Uninstalls a filter with given id. Should always be called when watch is no longer needed. Additonally Filters timeout when they aren't requested with shh_getFilterChanges for a period of time.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"shh_uninstallFilter",
"params":[
"0x7"
],
"id":73
}'
getFilterChanges - Polling method for whisper filters. Returns new messages since the last call of this method.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"shh_getFilterChanges",
"params":[
"0x7"
],
"id":73
}'
getMessages - Get all messages matching a filter. Unlike shh_getFilterChanges
this returns all messages.
curl --location --request POST 'localhost:8545/' \
--header 'Content-Type: application/json' \
--data-raw '{
"jsonrpc":"2.0",
"method":"shh_getMessages",
"params":[
"0x7"
],
"id":73
}'